Updating an existing short URL's slug
Update original URL, title or path for an existing short link by id
The instructions below demonstrate how to edit a slug (or the end part of a URL after the backslash “/” that identifies specific page or post) of an existing short URL. This user guide can be utilized to modify additional parameters (see Body params).
Body Params
path | optional path part of newly created link. If empty - it will be generated automatically | string |
title | title of created URL to be shown in Short.io admin panel | string |
tags | array of strings | |
expiresAt | Link expiration date, optional. We support Unix timestamp in milliseconds and ISO date/time format values If no expiration date is given (default), link will never expire | milliseconds since the epoch started (Unix) or yyyymmddThhmmss format (ISO date/time) |
expiredURL | URL to redirect when the link is expired | url |
iphoneURL | If users open the URL with iPhone, they will be redirected to this URL | url |
androidURL | If users open the URL with Android, they will be redirected to this URL | url |
password | Requires Personal plan. Password to be asked when user visits a link. This password will not be stored in plain text, we will hash it with salt | password |
utmSource, utmMedium, utmCampaign, utmTerm, utmContent | string | |
cloaking | double |
To update an existing short link
- Create a secret API key from the Integrations and API menu: https://app.short.io/settings/integrations/api-key
- Get the ID of the short link which you want to edit:
- In the Short.io Dashboard open the link for editing:

- Copy the link ID from your browser's address bar:
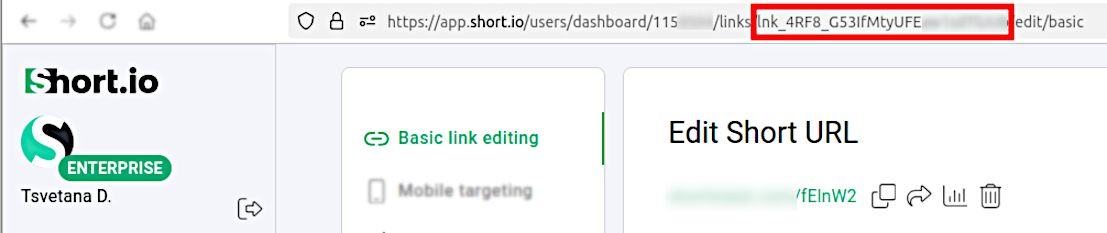
- Install prerequisites for requests (if necessary, depending on your programming language):
pip install requests
- Using the code snippet below, create a file: filename.py/ .js/ .rb
Please replace example.com , YOUR_PATH (the new slug to be applied), APIKEY and LINK_ID with the appropriate values.
import requests
url = "https://api.short.io/links/LINK_ID"
import json
payload = json.dumps({"allowDuplicates": False, "domain": "YOUR_DOMAIN", "path": "YOUR_PATH" })
headers = {
'accept': "application/json",
'content-type': "application/json",
'authorization': "<<apiKey>>"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
const data = {
"allowDuplicates":false,
"domain":"<<domain_name>>",
"path":"YOUR_PATH"
};
const options = {
headers: {
accept: 'application/json',
'content-type': 'application/json',
authorization: '<<apiKey>>'
},
method: "post",
body: JSON.stringify(data)
};
const response = await fetch('https://api.short.io/links/LINK_ID', options)
console.log(await response.json());
require 'uri'
require 'net/http'
require 'openssl'
require 'json'
url = URI("https://api.short.io/links/LINK_ID")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request["authorization"] = '<<apiKey>>'
request.body = JSON.generate({"allowDuplicates":false, "domain":"YOUR_DOMAIN", "path":"YOUT_PATH"})
response = http.request(request)
puts response.read_body
- Launch the file:
python filename.py
node filename.js
ruby filename.rb
- Review the JSON response:
{
originalURL: 'YOUR_LONG_LINK',
path: 'YOUR_PATH',
idString: 'LINK_ID',
id: 'LINK_ID',
shortURL: 'https://example.com/YOUR_PATH',
secureShortURL: 'https://example.com/YOUR_PATH',
cloaking: false,
tags: [],
createdAt: '2025-04-28T13:51:04.721Z',
skipQS: false,
archived: false,
DomainId: DOMAIN_ID,
OwnerId: OWNER_ID,
hasPassword: false,
source: 'api',
User: {
id: USER_ID,
name: 'YOUR_NAME',
email: 'YOUR_EMAIL_ADDRESS',
photoURL: 'YOUR_EMAIL_ADDRESS/PHOTO_ID'
}
}
You have successfully renamed a short link through our API:
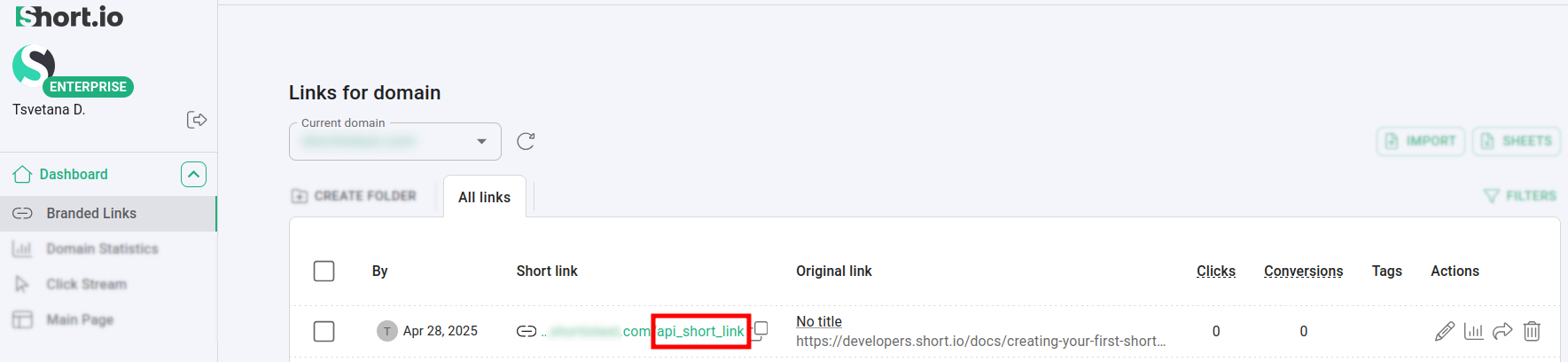
Most important keys in the response are PATH (the path or slug of the edited short link) and LINK_ID.
Updated 6 days ago