Unarchiving a short URL
This guide will assist you in restoring a previously archived short link.
To unarchive a short link
- Create a secret API key from the Integrations and API menu: https://app.short.io/settings/integrations/api-key
- Get the ID of the short link which you want to unarchive:
- In the Short.io Dashboard open the archived link for editing:

- Copy the link ID from your browser's address bar:
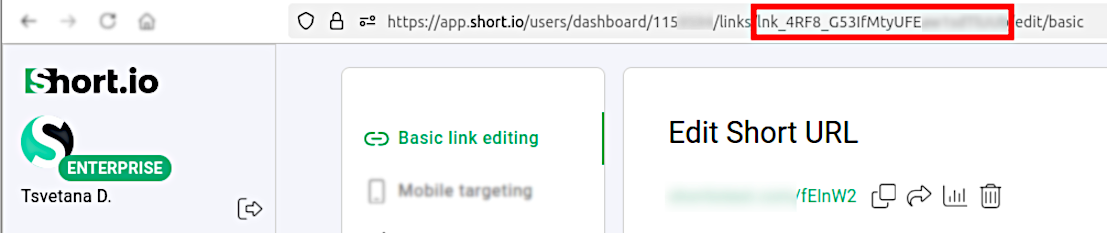
- Then you may need to install prerequisites for HTTP requests (if necessary, depending on your programming language and its version).
- Use the following code snippets to unarchive the short URL:
Please replace LINK_ID and APIKEY with the appropriate values.
<?php
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://api.short.io/links/unarchive",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => json_encode([
'link_id' => '<<link_id>>>>'
]),
CURLOPT_HTTPHEADER => [
"Authorization: <<apiKey>>",
"accept: application/json",
"content-type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
import requests
url = "https://api.short.io/links/unarchive"
payload = { "link_id": "<<link_id>>" }
headers = {
"accept": "application/json",
"content-type": "application/json",
"Authorization": "<<apiKey>>"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
const url = 'https://api.short.io/links/unarchive';
const options = {
method: 'POST',
headers: {
accept: 'application/json',
'content-type': 'application/json',
Authorization: '<<apiKey>>'
},
body: JSON.stringify({link_id: '<<link_id>>'})
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error(err));
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.short.io/links/unarchive"))
.header("accept", "application/json")
.header("content-type", "application/json")
.header("Authorization", "<<apiKey>>")
.method("POST", HttpRequest.BodyPublishers.ofString("{\"link_id\":\"<<link_id>>\"}"))
.build();
HttpResponse<String> response = HttpClient.newHttpClient().send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
using System.Net.Http.Headers;
var client = new HttpClient();
var request = new HttpRequestMessage
{
Method = HttpMethod.Post,
RequestUri = new Uri("https://api.short.io/links/unarchive"),
Headers =
{
{ "accept", "application/json" },
{ "Authorization", "<<apiKey>>" },
},
Content = new StringContent("{\"link_id\":\"<<link_id>>\"}")
{
Headers =
{
ContentType = new MediaTypeHeaderValue("application/json")
}
}
};
using (var response = await client.SendAsync(request))
{
response.EnsureSuccessStatusCode();
var body = await response.Content.ReadAsStringAsync();
Console.WriteLine(body);
}
- The JSON response should be "success": true:
{
"success":true
}
You have successfully unarchived a short link through our API.
The link is again visible from the Branded links panel.
And if you wish to unarchive several short links at the same time, please refer to the following webpage for more information: https://developers.short.io/reference/post_links-unarchive-bulk
Updated about 2 months ago