Adding Link Expiration to an existing short URL
This method creates an expiration date for an existing short URL
The instruction below shows how to create an expiration date for an existing short URL.
1) Get your secret API key here: https://app.short.io/settings/integrations/api-key
- Click "Create API key".
- Add a Secret key.
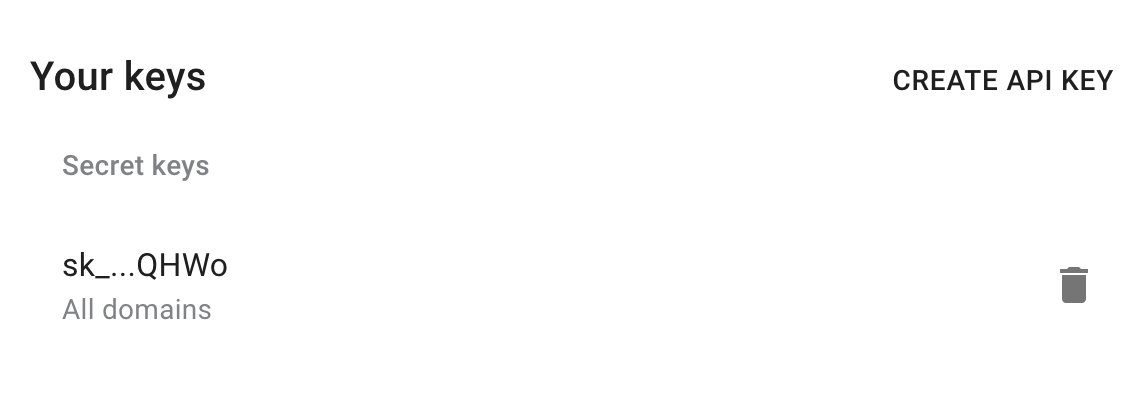
2) Copy an ID of a short link you want to edit.
- Open the statistics of the short link.
- Copy the link ID.
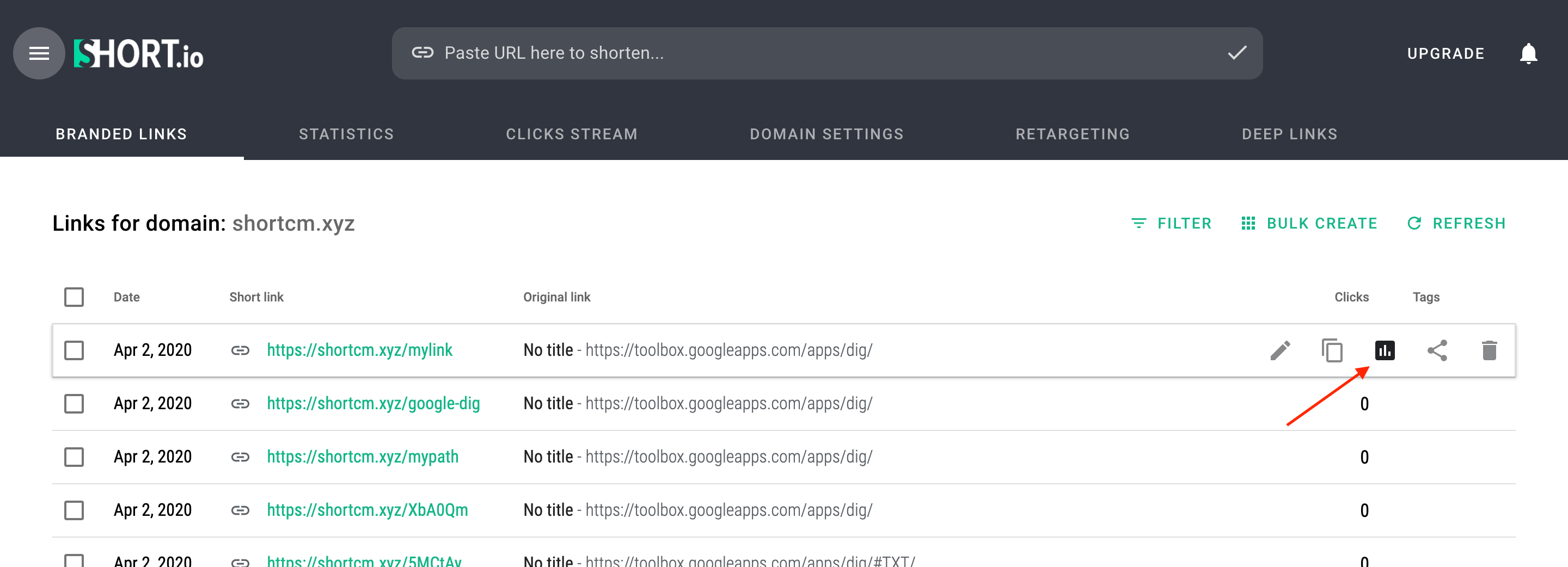
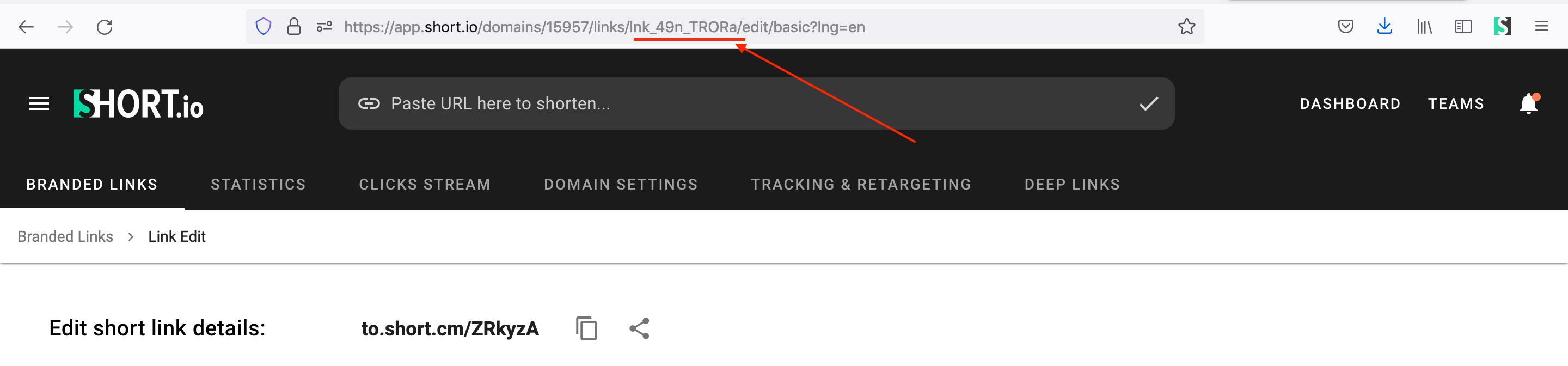
3) Install prerequisites for requests.
pip install requests
npm install --save axios
Now everything is ready to run the following snippet. It will create an expiration date and an expired URL for an existing short link.
4) Create a file: filename.py/ .js/ .rb. Use the code snippet below.
Please, replace LINK_ID, expiresAt and expiredURL with appropriate values.
import requests
import time
import datetime
url = "https://api.short.io/links/LINK_ID"
import json
payload = json.dumps({"expiredURL":"https://analytics.google.com/analytics/web/", "expiresAt": time.mktime(datetime.datetime.strptime("22.05.2020 15:56:53", "%d.%m.%Y %H:%M:%S").timetuple()) * 1000 })
headers = {
'accept': "application/json",
'content-type': "application/json",
'authorization': "<<apiKey>>"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
const axios = require('axios');
const data = {
"expiredURL":"https://analytics.google.com/analytics/web/",
"expiresAt":new Date("2020-05-22T15:56:53").valueOf()
};
const options = {
headers: {
accept: 'application/json',
'content-type': 'application/json',
authorization: '<<apiKey>>'
}
};
axios.post('https://api.short.io/links/LINK_ID', data, options)
.then(function (response) {
console.log(response.data);
})
.catch(function (response) {
console.log(response);
});
require 'uri'
require 'net/http'
require 'openssl'
require 'json'
require 'time'
url = URI("https://api.short.io/links/LINK_ID")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request["authorization"] = '<<apiKey>>'
request.body = JSON.generate({"expiredURL":"https://analytics.google.com/analytics/web/", "expiresAt": Time.parse("22.05.2020 15:56:53").to_i * 1000})
response = http.request(request)
puts response.read_body
5) Launch the file.
python filename.py
node filename.js
ruby filename.rb
6) JSON Response (link expiration is created).
Once you run the code, you will see the response.
{
id: 280142679,
path: 'CvCZHC',
title: 'Link queries',
icon: 'https://shortcm-icons.s3.us-west-2.amazonaws.com/14ea9cc5e8eb3895967d9461f8e170d5',
archived: false,
originalURL: 'https://developers.short.cm/reference#linksbylinkidpost',
iphoneURL: null,
androidURL: null,
splitURL: null,
expiresAt: '2020-05-22T12:56:53.000Z',
expiredURL: 'https://analytics.google.com/analytics/web/',
redirectType: null,
cloaking: null,
source: 'slack',
AutodeletedAt: null,
createdAt: '2020-04-24T07:50:37.000Z',
updatedAt: '2020-04-27T07:09:25.982Z',
DomainId: 902,
OwnerId: 934,
tags: [],
secureShortURL: 'https://shortc.xyz/CvCZHC',
shortURL: 'https://shortc.xyz/CvCZHC'
}
Here's how it looks on Short.io:
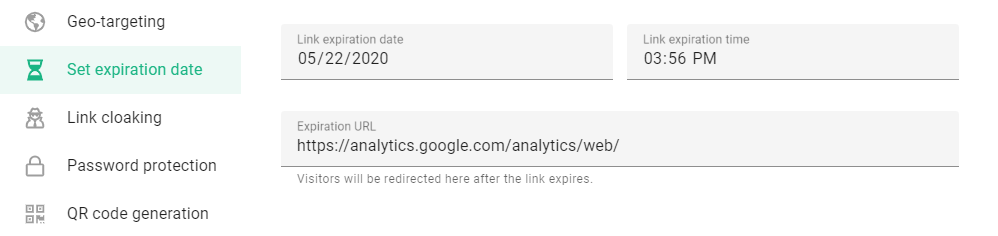
Updated almost 4 years ago